https://nodejs.org/en
- Node.js LTS sürümü tavsiye edilir, nasıl kurulum yapıldığında youtube'dan bakabilirsiniz.
- Node.js'i kurduktan sonra masaüstünde SHIFT + SAĞ TIK yaptıktan sonra PowerShell penceresini açıyoruz.
- Açılan pencereye npm install axios komutunu yapıştırıp kütüphanenin yüklenmesini bekliyoruz.
- Ekte yer alan javascript kodlarını bot.js adlı dosya ile masaüstüne kaydediniz.
- Masaüstünde açık olan PowerShell penceresine node bot.js komutunu yazın.
- Bot çalışmaya başladı, başarılı sitelerde yeşil şekilde ekrana domain ve başarılı mesajı düşecektir.
Yorum olarak siteye gönderilen veri içeriğini 139. satırdan düzenleyebilirsiniz.
Backlink listesini links.txt adlı TXT dosyası içerisinde masaüstünde bot.js ile aynı konumda bulundurmanız gerekiyor.
Botu durdurmak için powershell penceresini kapatmanız yeterlidir.
127. ve 131. satırlarındaki yorum satırlarını devredışı bırakarak array olarak detaylı çıktı alabilirsiniz. Ekranın çok dolmaması için sadece başarılı işlemleri konsol'a işleyecek şekilde kodu düzenledik.
İşlem yapılan backlinkler links.txt dosyasından silinmektedir, bu nedenle TXT'de link kalmadığında bot otomatik olarak duracaktır.
Maksimum 60MB ram kullanımı yapmaktadır.
Satışı yasaktır, ücretsiz dağıtılmalıdır.
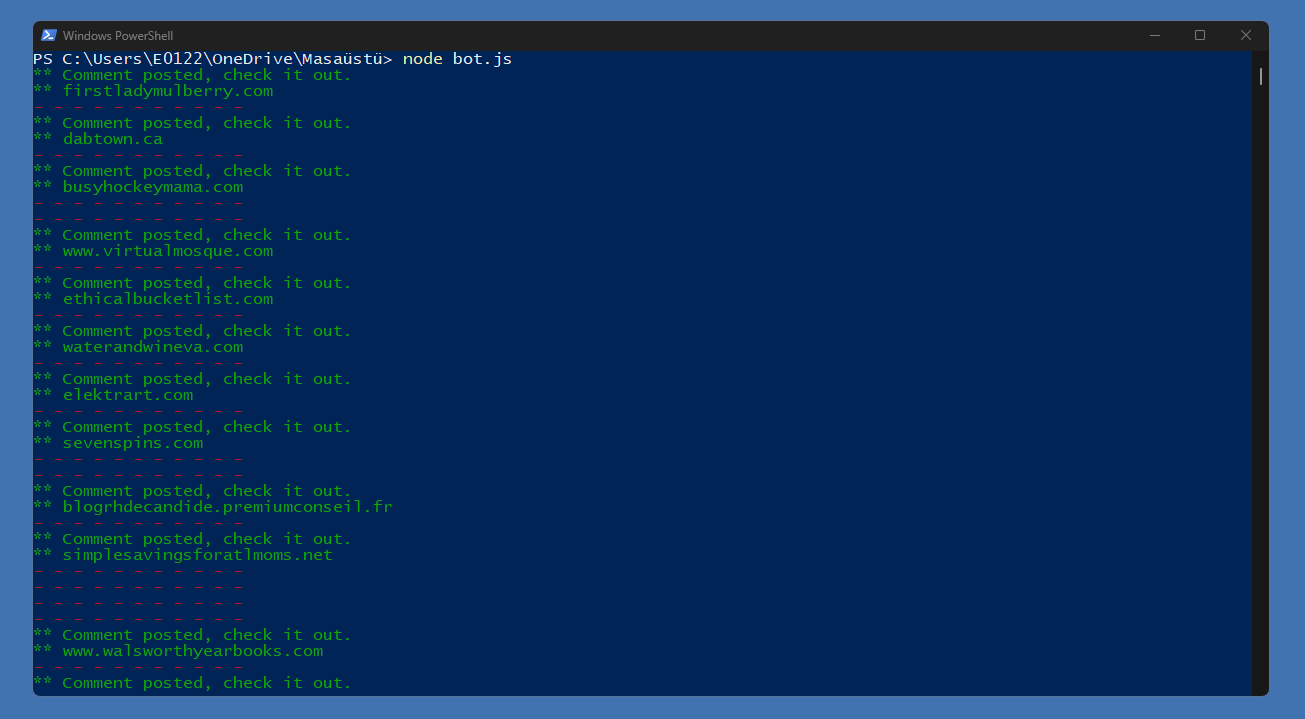
const axios = require('axios'); const fs = require('fs').promises; class WpCommentBot { static async basicAxios(address) { try { const response = await axios.get(address, { timeout: 60000, // 60 saniye headers: { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/87.0.4280.141 Safari/537.36' } }); return { response: response.data, http_status: response.status }; } catch (error) { if (error.response) { // Hata, HTTP durum koduyla birlikte geldi // console.error(`Error making GET request to ${address}: HTTP Status ${error.response.status}`); return { response: '', http_status: error.response.status }; } else { // console.error(`Error making GET request to ${address}: ${error.message}`); return { response: '', http_status: 0 }; } } } static async findWpCommentsPost(url) { const exUrl = url.split('/'); const mainTest = await this.basicAxios(`https://${new URL(url).host}/wp-comments-post.php`); const firstDirectoryTest = await this.basicAxios(`https://${exUrl[2]}/${exUrl[3]}/wp-comments-post.php`); if (mainTest.http_status === 405) { return `https://${new URL(url).host}/wp-comments-post.php`; } else if (firstDirectoryTest.http_status === 405) { return `https://${exUrl[2]}/${exUrl[3]}`; } return false; } static async sendComment(postAddress, variables = {}) { const { comment, author, email, site_address } = variables; const { response, http_status } = await this.basicAxios(postAddress); const post_idMatch = response.match(/<input type='hidden' name='comment_post_ID' value='(.*?)'/si); if (!post_idMatch) { return { status: 0, comment_address: postAddress, message: `${new URL(postAddress).host} could not find post_id data of the address` }; } const post_id = post_idMatch[1]; const postAddressCurl = await this.findWpCommentsPost(postAddress); if (postAddressCurl == false ) { return { status: 0, comment_address: postAddress, message: `${new URL(postAddress).host} could not find wp-comments-post.php of the address` }; } const postFields = { comment, author, email, url: site_address, comment_post_ID: post_id, comment_parent: 0 }; try { const response = await axios.post(postAddressCurl, postFields, { timeout: 60000, // 60 saniye headers: { 'User-Agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_11_4) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/51.0.2704.79 Safari/537.36', 'Referer': postAddress } }); if (response.data.includes("error-page")) { return { status: 0, comment_address: postAddress, message: "You have posted the same comment before or have been blocked by this site. Failed to submit comment" }; } else if (response.data.includes("comment-awaiting-moderation")) { return { status: 1, comment_address: postAddress, message: "The comment probably fell into the approval process" }; } else { console.log('\x1b[32m%s\x1b[0m', `** Comment posted, check it out.`); console.log('\x1b[32m%s\x1b[0m', `** ${new URL(postAddress).host}`); return { status: 1, comment_address: postAddress, message: "Comment posted, check it out" }; } } catch (error) { //console.error(`Error making POST request to ${postAddressCurl}: ${error.message}`); return { status: 0, comment_address: postAddress, message: "Failed to submit comment" }; } } static async sendCommentMultiple(postAddresses, variables = {}) { // const outputs = []; for (const postAddress of postAddresses) { const sendComment = await this.sendComment(postAddress, variables); //outputs.push(sendComment); } console.log('\x1b[31m%s\x1b[0m', '- - - - - - - - - - -'); } } const commentVariables = { comment: 'Yorum içeriği', author: 'Yorum Ad Soyad', email: 'Yorum mail adresi', site_address: 'Site linkiniz' }; async function main() { try { const linksTxt = await fs.readFile('links.txt', 'utf8'); const links = linksTxt.trim().split('\n'); for (const originalLink of links) { const link = originalLink.replace(/\r/g, ''); // \r karakterlerini temizle const postAddresses = [link]; const results = await WpCommentBot.sendCommentMultiple(postAddresses, commentVariables); const updatedLinks = links.filter(l => l !== originalLink); // Temizlenmemiş linki kaldır await fs.writeFile('links.txt', updatedLinks.join('\n'), 'utf8'); } } catch (error) { // Hata durumunu işleme alabilirsiniz console.error('An error occurred:', error); } } main();PHP ile stabil şekilde çalışmadığı için aşağıdaki kütüphanenin NODE.JS'e çevrilmiş halidir.
https://github.com/v4r1able/wordpres...-bot/tree/main