Outlook Desteği Servisimize Eklenmiştir.

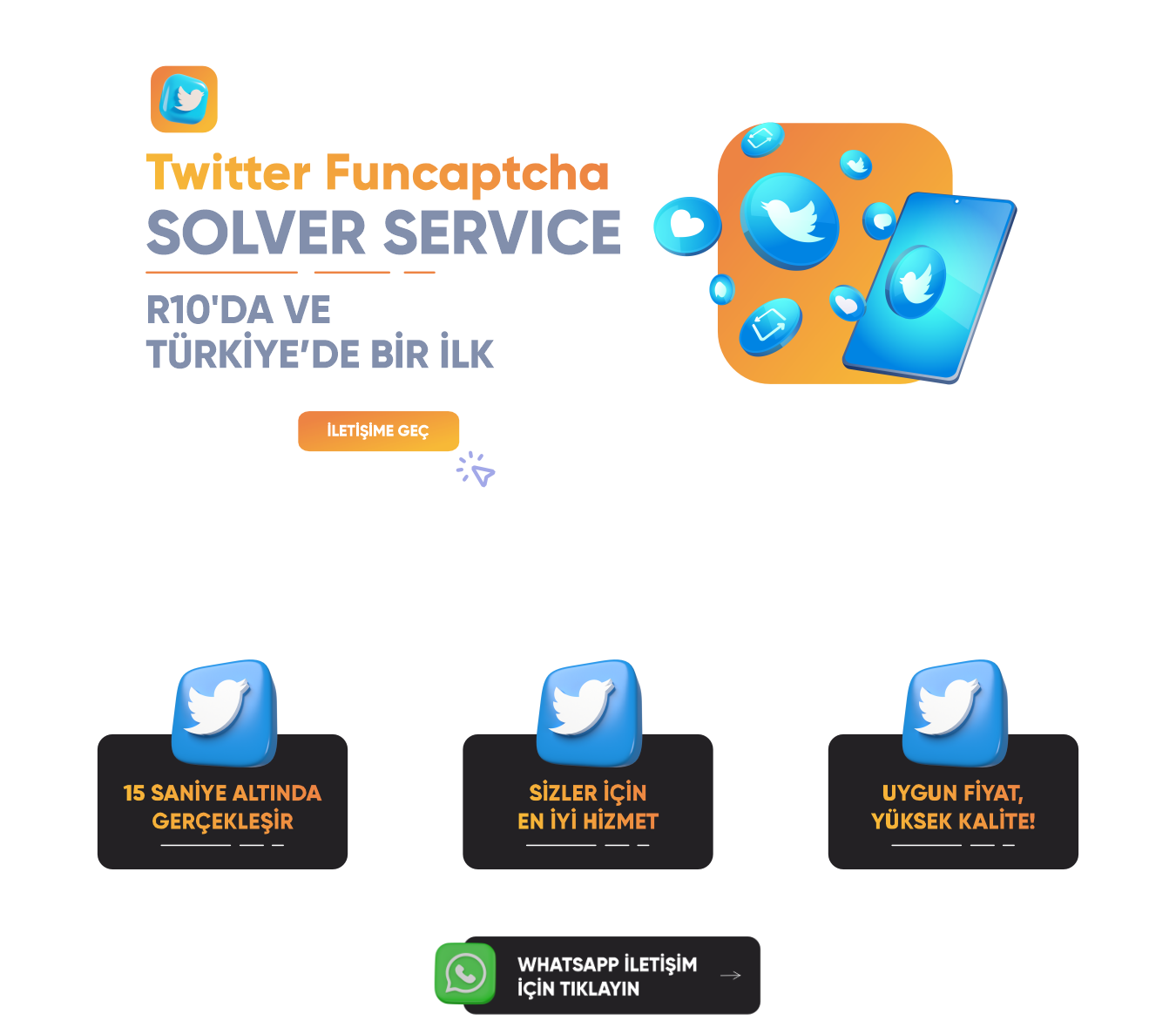
Fiyat : 1K/3$ Sabitlenmiştir.

0152B4EB-D2DC-460A-89A1-629838B529C9
2CB16598-CB82-4CF7-B332-5990DB66F3AB
867D55F2-24FD-4C56-AB6D-589EDAF5E7C5
B7D8911C-5CC8-A9A3-35B0-554ACEE604DA
2CB16598-CB82-4CF7-B332-5990DB66F3AB
867D55F2-24FD-4C56-AB6D-589EDAF5E7C5
B7D8911C-5CC8-A9A3-35B0-554ACEE604DA

using System; using System.Net.Http; using System.Threading.Tasks; using Newtonsoft.Json.Linq; public class FunCaptcha { // Asenkron GET isteği gönderen metot static async Task<string> SendGetRequest(string url) { using (HttpClient client = new HttpClient()) { HttpResponseMessage response = await client.GetAsync(url); if (response.IsSuccessStatusCode) { return await response.Content.ReadAsStringAsync(); } else { Console.WriteLine($"Error: {response.StatusCode} - {response.ReasonPhrase}"); return null; } } } // Captcha token'ını almak için ana metot public async Task<string> GetCaptcha(string apiKey, string funCaptchaSiteKey) { string result = ""; int maxAttempts = 60; // Maksimum deneme sayısı int attempts = 0; // Belirtilen maksimum deneme sayısına ulaşana kadar veya başarısız olana kadar döngü while (attempts < maxAttempts) { // Captcha görevini oluşturmak için FunCaptcha API'ye GET isteği gönder string createTaskingUrl = $"https://fun.vocopus.com/FunCaptcha?key={apiKey}&FunCaptchaSiteKey={funCaptchaSiteKey}"; string createTaskingResponse = await SendGetRequest(createTaskingUrl); JObject jsonResponse = JObject.Parse(createTaskingResponse); // Hata kontrolü if (jsonResponse["error"] != null && jsonResponse["error"].ToString() == "Yetersiz bakiye") { Console.WriteLine("Hata: Yetersiz bakiye. İşlemler iptal edildi."); return "Yetersiz bakiye"; } if (jsonResponse["error"] != null && jsonResponse["error"].ToString() == "Kullanıcı bulunamadı") { Console.WriteLine("Hata: Api Key Hatalı"); return "Api Key Hatalı"; } string orderIDValue = jsonResponse["orderID"]?.ToString(); // Oluşturulan görev başarılıysa, görevin durumunu kontrol et if (!string.IsNullOrEmpty(orderIDValue)) { while (true) { string orderID = orderIDValue; string checkTaskingUrl = $"https://fun.vocopus.com/FunCaptcha?key={apiKey}&orderID={orderID}"; // Captcha görevinin durumunu kontrol etmek için FunCaptcha API'ye GET isteği gönder string checkTaskingResponse = await SendGetRequest(checkTaskingUrl); JObject checkTaskingJson = JObject.Parse(checkTaskingResponse); string status = checkTaskingJson["order"]?["status"]?.ToString(); // Görev başarılıysa, captcha token'ını al ve döngüyü sonlandır if (status == "Success") { string token = checkTaskingJson["order"]?["token"].ToString(); result = token; Console.WriteLine("Success! Exiting the loop."); return result; } // Görev başarısızsa, döngüyü sonlandır else if (status == "Fail") { Console.WriteLine("Fail status received. Exiting the loop."); break; } // Belirli bir süre beklet (isteğe bağlı olarak değiştirilebilir) await Task.Delay(1000); } } attempts++; // Belirli bir süre beklet (isteğe bağlı olarak değiştirilebilir) await Task.Delay(1000); } // Maksimum deneme sayısına ulaşıldığında veya hata durumunda sonuç return result; } // Uygulamanın giriş noktası public static async Task Main(string[] args) { // API anahtarınızı ve Twitter site anahtarınızı girin string apiKey = "Your_Api_Key"; string twitterSiteKey = "2CB16598-CB82-4CF7-B332-5990DB66F3AB"; // FunCaptcha sınıfından bir örnek oluşturun FunCaptcha funCaptcha = new FunCaptcha(); // Captcha token'ını al string captchaResponse = await funCaptcha.GetCaptcha(apiKey, twitterSiteKey); // Captcha token'ını kullanarak başka işlemler yapabilirsiniz // ... } }

<?php class FunCaptcha { private function sendGetRequest($url) { $client = new GuzzleHttpClient(); $response = $client->get($url); if ($response->getStatusCode() == 200) { return $response->getBody()->getContents(); } else { echo "Error: " . $response->getStatusCode() . " - " . $response->getReasonPhrase() . PHP_EOL; return null; } } public function getCaptcha($apiKey, $funCaptchaSiteKey) { $result = ""; $maxAttempts = 60; $attempts = 0; while ($attempts < $maxAttempts) { $createTaskingUrl = "https://fun.vocopus.com/FunCaptcha?key=$apiKey&FunCaptchaSiteKey=$funCaptchaSiteKey"; $createTaskingResponse = $this->sendGetRequest($createTaskingUrl); $jsonResponse = json_decode($createTaskingResponse, true); if (isset($jsonResponse["error"]) && $jsonResponse["error"] == "Yetersiz bakiye") { echo "Hata: Yetersiz bakiye. İşlemler iptal edildi." . PHP_EOL; return "Yetersiz bakiye"; } if (isset($jsonResponse["error"]) && $jsonResponse["error"] == "Kullanıcı bulunamadı") { echo "Hata: Api Key Hatalı" . PHP_EOL; return "Api Key Hatalı"; } $orderIDValue = $jsonResponse["orderID"] ?? null; if (!empty($orderIDValue)) { while (true) { $orderID = $orderIDValue; $checkTaskingUrl = "https://fun.vocopus.com/FunCaptcha?key=$apiKey&orderID=$orderID"; $checkTaskingResponse = $this->sendGetRequest($checkTaskingUrl); $checkTaskingJson = json_decode($checkTaskingResponse, true); $status = $checkTaskingJson["order"]["status"] ?? null; if ($status == "Success") { $token = $checkTaskingJson["order"]["token"]; $result = $token; echo "Success! Exiting the loop." . PHP_EOL; return $result; } elseif ($status == "Fail") { echo "Fail status received. Exiting the loop." . PHP_EOL; break; } // Belirli bir süre beklet (isteğe bağlı olarak değiştirilebilir) sleep(1); } } $attempts++; // Belirli bir süre beklet (isteğe bağlı olarak değiştirilebilir) sleep(1); } // Maksimum deneme sayısına ulaşıldığında veya hata durumunda sonuç return $result; } } // Uygulamanın giriş noktası $apiKey = "Your_Api_Key"; $twitterSiteKey = "2CB16598-CB82-4CF7-B332-5990DB66F3AB"; // FunCaptcha sınıfından bir örnek oluşturun $funCaptcha = new FunCaptcha(); // Captcha token'ını al $captchaResponse = $funCaptcha->getCaptcha($apiKey, $twitterSiteKey); // Captcha token'ını kullanarak başka işlemler yapabilirsiniz // ... ?>

import asyncio import aiohttp import json import time class FunCaptcha: async def send_get_request(self, url): async with aiohttp.ClientSession() as session: async with session.get(url) as response: if response.status == 200: return await response.text() else: print(f"Error: {response.status} - {response.reason}") return None async def get_captcha(self, api_key, fun_captcha_site_key): result = "" max_attempts = 60 attempts = 0 while attempts < max_attempts: create_tasking_url = f"https://fun.vocopus.com/FunCaptcha?key={api_key}&FunCaptchaSiteKey={fun_captcha_site_key}" create_tasking_response = await self.send_get_request(create_tasking_url) json_response = json.loads(create_tasking_response) if "error" in json_response and json_response["error"] == "Yetersiz bakiye": print("Hata: Yetersiz bakiye. İşlemler iptal edildi.") return "Yetersiz bakiye" if "error" in json_response and json_response["error"] == "Kullanıcı bulunamadı": print("Hata: Api Key Hatalı") return "Api Key Hatalı" order_id_value = json_response.get("orderID") if order_id_value: while True: order_id = order_id_value check_tasking_url = f"https://fun.vocopus.com/FunCaptcha?key={api_key}&orderID={order_id}" check_tasking_response = await self.send_get_request(check_tasking_url) check_tasking_json = json.loads(check_tasking_response) status = check_tasking_json.get("order", {}).get("status") if status == "Success": token = check_tasking_json.get("order", {}).get("token") result = token print("Success! Exiting the loop.") return result elif status == "Fail": print("Fail status received. Exiting the loop.") break await asyncio.sleep(1) attempts += 1 await asyncio.sleep(1) return result async def main(): api_key = "Your_Api_Key" twitter_site_key = "2CB16598-CB82-4CF7-B332-5990DB66F3AB" fun_captcha = FunCaptcha() captcha_response = await fun_captcha.get_captcha(api_key, twitter_site_key) # Captcha token'ını kullanarak başka işlemler yapabilirsiniz # ... if name == "main": asyncio.run(main())